Download Mstest Driver
Unity is the ultimate real-time 2D, 3D, AR, & VR development engine. Download Unity to start creating today and get access to the Unity platform and ecosystem. Chrome WebDriver (make sure the driver version matches the browser version) 1. Creating the.NET Core test project # mkdir SeleniumDotNet cd SeleniumDotNet dotnet new solution mkdir Demo.SeleniumTests cd Demo.SeleniumTests dotnet new mstest cd. Dotnet sln add Demo.SeleniumTests Using the above commands, the skeleton of your test project is.
Selenium is a browser automation tool mainly used for UI testing and automating tasks. Selenium is agnostic of operating system, programming language, and browser.
You can automate Chrome on MacOS using C#, FireFox using Python on Windows, or Opera using NodeJS on Linux to give you a few examples. Refer to this page on the Selenium website for a full list of supported OS's, programming languages, and browsers.
In this post you'll learn how to create a minimal Selenium test using .NET Core on both Windows and Ubuntu (Linux).
FYI, this post should also apply for MacOS, though I have not verified this. To follow along you'll need the following:
- .NET Core SDK (2.2)
- Windows, Linux, or MacOS dev machine
- Chrome/Chromium web browser
- Chrome WebDriver (make sure the driver version matches the browser version)
1. Creating the .NET Core test project #
Using the above commands, the skeleton of your test project is ready. Navigate to your test project and run the following command to test out the dummy test:
2. Add Selenium to the test project #
To start integrating Selenium into your test project, you'll need to add the Selenium.WebDriver Nuget package.
Use the following dotnet cli command to add the dependency.
Once the package has been added, you'll be able to use the Selenium API in your C# project but you'll need to make the ChromeDriver available before it'll start working. More on that later.
3. Write a UI test using Selenium #
Using your editor of choice, write a UI test. Keep it simple for now, you can write a more advanced test after getting a simple test working.
Here's a more advanced sample, but feel free to strip it to the minimum:
Note: The test is likely to break when the dotnet site changes.

Pay attention to the constructor of 'ChromeDriver' on line 16. By default the ChromeDriver class when instantiated without parameters will search forβ the chromedriver executable (from chromedriver.chromium.org) inside your Path environment variable.
Alternatively, you can pass in the path of the ChromeDriver executable to the constructor. By passing in '.', Selenium will search for the executable in the current working directory.
4. Run the UI test #
To run the test follow these steps:
- Run the dotnet build command inside your test project
- Copy the chromedriver.exe/chromedriver executable to 'Selenium_DotNetDemo.SeleniumTestsbinDebugnetcoreapp2.2β'
See example scripts below for Ubuntu and PowerShell. - Run the dotnet test command and watch the magic π§ββοΈ
For Ubuntu you can use these commands to download the ChromeDriver (modify driver URL as needed):
For Windows you can use this PowerShell script (modify driver URL as needed):
Troubleshooting: if the test fails due to Chrome/Chromium errors, follow these steps
- Make sure you have Chrome/Chromium installed.
- Make sure the ChromeDriver supports your version of Chrome/Chromium
- Make sure you have the permissions to execute the ChromeDriver executable
- If your OS is shell based without any GUI, you have to use Chrome in headless mode.
This snippet shows how to start the ChromeDriver+Chrome in headless mode.
Summary #

Using .NET Core you can write cross-platform UI tests using C# and Selenium. You can swap out the ChromeDriver with any other supported browser to verify cross-browser compatibility. You can use this GitHub repository as a reference in case you run into any roadblocks.
In the next post, learn how you can run these UI tests inside of Azure DevOps deployment pipelines.
Cheers π₯
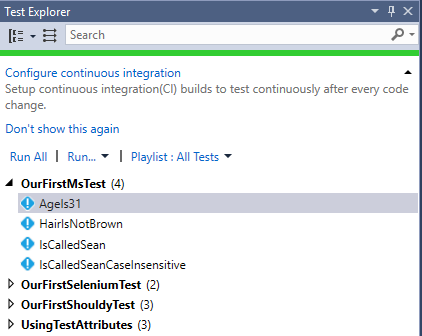
Notes:
- The reason I don't suggest using Nuget packages to pull in the ChromeDriver executable is because executable is only offered by 3rd party unverified package owners which introduces a risky dependency into your codebase.
- I suggest not including the ChromeDriver executable in your .csproj file or Source Control since there's no guarantee ahead of time that your Chrome browser will be compatible with your ChromeDriver. For example, when you pass this test to a test server, the Chrome browser may be on a version incompatible with your ChromeDriver. Some solutions to this would be to:
- include the ChromeDriver in the path environment variable of the test server;
- download the correct ChromeDriver as part of your CI/CD system and copy it next to your test dll's;
- pass in the location of the ChromeDriver through TestRunParameters;
This article applies to: βοΈ .NET Core 2.1 SDK and later versions
Name
dotnet test
- .NET test driver used to execute unit tests.
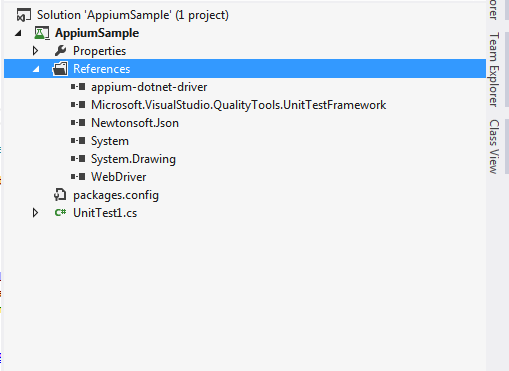
Synopsis
Description
The dotnet test
command is used to execute unit tests in a given solution. The dotnet test
command builds the solution and runs a test host application for each test project in the solution. The test host executes tests in the given project using a test framework, for example: MSTest, NUnit, or xUnit, and reports the success or failure of each test. If all tests are successful, the test runner returns 0 as an exit code; otherwise if any test fails, it returns 1.
For multi-targeted projects, tests are run for each targeted framework. The test host and the unit test framework are packaged as NuGet packages and are restored as ordinary dependencies for the project.
Test projects specify the test runner using an ordinary <PackageReference>
element, as seen in the following sample project file:
Where Microsoft.NET.Test.Sdk
is the test host, xunit
is the test framework. And xunit.runner.visualstudio
is a test adapter, which allows the xUnit framework to work with the test host.
Implicit restore
You don't have to run dotnet restore
because it's run implicitly by all commands that require a restore to occur, such as dotnet new
, dotnet build
, dotnet run
, dotnet test
, dotnet publish
, and dotnet pack
. To disable implicit restore, use the --no-restore
option.
The dotnet restore
command is still useful in certain scenarios where explicitly restoring makes sense, such as continuous integration builds in Azure DevOps Services or in build systems that need to explicitly control when the restore occurs.

For information about how to manage NuGet feeds, see the dotnet restore
documentation.
Arguments
PROJECT | SOLUTION | DIRECTORY | DLL
- Path to the test project.
- Path to the solution.
- Path to a directory that contains a project or a solution.
- Path to a test project .dll file.
If not specified, it searches for a project or a solution in the current directory.
Options
-a|--test-adapter-path <ADAPTER_PATH>
Path to a directory to be searched for additional test adapters. Only .dll files with suffix
.TestAdapter.dll
are inspected. If not specified, the directory of the test .dll is searched.--blame
Runs the tests in blame mode. This option is helpful in isolating problematic tests that cause the test host to crash. When a crash is detected, it creates a sequence file in
TestResults/<Guid>/<Guid>_Sequence.xml
that captures the order of tests that were run before the crash.--blame-crash
(Available since .NET 5.0 preview SDK)Runs the tests in blame mode and collects a crash dump when the test host exits unexpectedly. This option depends on the version of .NET used, the type of error, and the operating system.
For exceptions in managed code, a dump will be automatically collected on .NET 5.0 and later versions. It will generate a dump for testhost or any child process that also ran on .NET 5.0 and crashed. Crashes in native code will not generate a dump. This option works on Windows, macOS, and Linux.
Crash dumps in native code, or when using .NET Core 3.1 or earlier versions, can only be collected on Windows, by using Procdump. A directory that contains procdump.exe and procdump64.exe must be in the PATH or PROCDUMP_PATH environment variable. Download the tools. Implies
--blame
.To collect a crash dump from a native application running on .NET 5.0 or later, the usage of Procdump can be forced by setting the
VSTEST_DUMP_FORCEPROCDUMP
environment variable to1
.--blame-crash-dump-type <DUMP_TYPE>
(Available since .NET 5.0 preview SDK)The type of crash dump to be collected. Implies
--blame-crash
.--blame-crash-collect-always
(Available since .NET 5.0 preview SDK)Collects a crash dump on expected as well as unexpected test host exit.
--blame-hang
(Available since .NET 5.0 preview SDK)Run the tests in blame mode and collects a hang dump when a test exceeds the given timeout.
--blame-hang-dump-type <DUMP_TYPE>
(Available since .NET 5.0 preview SDK)The type of crash dump to be collected. It should be
full
,mini
, ornone
. Whennone
is specified, test host is terminated on timeout, but no dump is collected. Implies--blame-hang
.--blame-hang-timeout <TIMESPAN>
(Available since .NET 5.0 preview SDK)Per-test timeout, after which a hang dump is triggered and the test host process and all of its child processes are dumped and terminated. The timeout value is specified in one of the following formats:
- 1.5h, 1.5hour, 1.5hours
- 90m, 90min, 90minute, 90minutes
- 5400s, 5400sec, 5400second, 5400seconds
- 5400000ms, 5400000mil, 5400000millisecond, 5400000milliseconds
When no unit is used (for example, 5400000), the value is assumed to be in milliseconds. When used together with data driven tests, the timeout behavior depends on the test adapter used. For xUnit and NUnit the timeout is renewed after every test case. For MSTest, the timeout is used for all test cases. This option is supported on Windows with netcoreapp2.1 and later, on Linux with netcoreapp3.1 and later, and on macOS with net5.0 or later. Implies
--blame
and--blame-hang
.-c|--configuration <CONFIGURATION>
Defines the build configuration. The default value is
Debug
, but your project's configuration could override this default SDK setting.--collect <DATA_COLLECTOR_NAME>
Enables data collector for the test run. For more information, see Monitor and analyze test run.
To collect code coverage on any platform that is supported by .NET Core, install Coverlet and use the
--collect:'XPlat Code Coverage'
option.On Windows, you can collect code coverage by using the
--collect 'Code Coverage'
option. This option generates a .coverage file, which can be opened in Visual Studio 2019 Enterprise. For more information, see Use code coverage and Customize code coverage analysis.-d|--diag <LOG_FILE>
Enables diagnostic mode for the test platform and writes diagnostic messages to the specified file and to files next to it. The process that is logging the messages determines which files are created, such as
*.host_<date>.txt
for test host log, and*.datacollector_<date>.txt
for data collector log.-f|--framework <FRAMEWORK>
Forces the use of
dotnet
or .NET Framework test host for the test binaries. This option only determines which type of host to use. The actual framework version to be used is determined by the runtimeconfig.json of the test project. When not specified, the TargetFramework assembly attribute is used to determine the type of host. When that attribute is stripped from the .dll, the .NET Framework host is used.--filter <EXPRESSION>
Filters out tests in the current project using the given expression. For more information, see the Filter option details section. For more information and examples on how to use selective unit test filtering, see Running selective unit tests.
-h|--help
Prints out a short help for the command.
--interactive
Allows the command to stop and wait for user input or action. For example, to complete authentication. Available since .NET Core 3.0 SDK.
-l|--logger <LOGGER>
Specifies a logger for test results. Unlike MSBuild, dotnet test doesn't accept abbreviations: instead of
-l 'console;v=d'
use-l 'console;verbosity=detailed'
. Specify the parameter multiple times to enable multiple loggers.--no-build
Doesn't build the test project before running it. It also implicitly sets the -
--no-restore
flag.--nologo
Run tests without displaying the Microsoft TestPlatform banner. Available since .NET Core 3.0 SDK.
--no-restore
Doesn't execute an implicit restore when running the command.
-o|--output <OUTPUT_DIRECTORY>
Directory in which to find the binaries to run. If not specified, the default path is
./bin/<configuration>/<framework>/
. For projects with multiple target frameworks (via theTargetFrameworks
property), you also need to define--framework
when you specify this option.dotnet test
always runs tests from the output directory. You can use AppDomain.BaseDirectory to consume test assets in the output directory.-r|--results-directory <RESULTS_DIR>
The directory where the test results are going to be placed. If the specified directory doesn't exist, it's created. The default is
TestResults
in the directory that contains the project file.--runtime <RUNTIME_IDENTIFIER>
The target runtime to test for.
-s|--settings <SETTINGS_FILE>
The
.runsettings
file to use for running the tests. TheTargetPlatform
element (x86|x64) has no effect fordotnet test
. To run tests that target x86, install the x86 version of .NET Core. The bitness of the dotnet.exe that is on the path is what will be used for running tests. For more information, see the following resources:-t|--list-tests
List the discovered tests instead of running the tests.
-v|--verbosity <LEVEL>
Sets the verbosity level of the command. Allowed values are
q[uiet]
,m[inimal]
,n[ormal]
,d[etailed]
, anddiag[nostic]
. The default isminimal
. For more information, see LoggerVerbosity.RunSettings
arguments
Inline RunSettings
are passed as the last arguments on the command line after '-- ' (note the space after --). Inline RunSettings
are specified as [name]=[value]
pairs. A space is used to separate multiple [name]=[value]
pairs.
Example: dotnet test -- MSTest.DeploymentEnabled=false MSTest.MapInconclusiveToFailed=True
For more information, see Passing RunSettings arguments through command line.
Examples
Run the tests in the project in the current directory:
Run the tests in the
test1
project:Run the tests in the project in the current directory, and generate a test results file in the trx format:
Run the tests in the project in the current directory, and generate a code coverage file (after installing Coverlet collectors integration):
Run the tests in the project in the current directory, and generate a code coverage file (Windows only):
Run the tests in the project in the current directory, and log with detailed verbosity to the console:
Run the tests in the project in the current directory, and report tests that were in progress when the test host crashed:
Filter option details
--filter <EXPRESSION>
<Expression>
has the format <property><operator><value>[|&<Expression>]
.
<property>
is an attribute of the Test Case
. The following are the properties supported by popular unit test frameworks:
Test Framework | Supported properties |
---|---|
MSTest |
|
xUnit |
|
NUnit |
|
The <operator>
describes the relationship between the property and the value:
Operator | Function |
---|---|
= | Exact match |
!= | Not exact match |
~ | Contains |
!~ | Not contains |
<value>
is a string. All the lookups are case insensitive.
An expression without an <operator>
is automatically considered as a contains
on FullyQualifiedName
property (for example, dotnet test --filter xyz
is same as dotnet test --filter FullyQualifiedName~xyz
).
Expressions can be joined with conditional operators:
Operator | Function |
---|---|
| | OR |
& | AND |
You can enclose expressions in parenthesis when using conditional operators (for example, (Name~TestMethod1) | (Name~TestMethod2)
).
For more information and examples on how to use selective unit test filtering, see Running selective unit tests.
Download Mstest Drivers
See also
